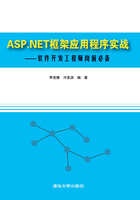
2.10 HTML及URL帮助器
绝大部分Web请求的目标都是向用户发送HTML代码。正因为如此,ASP.NET MVC会尽力帮助你去创建HTML。除了Razor标记语言,ASP.NET MVC也提供了很多生成HTML代码的既简单又有效的方式,比如提供了帮助类。最重要的两个帮助类就是HtmlHelper类和UrlHelper类,作为控制器和视图的HTML和URL属性暴露出来,供大家使用。
2.10.1 HTML帮助器
HtmlHelper类提供了一些方法,帮助用户以编程方式创建HTML控件。所有HtmlHelper方法均生成HTML,并以字符串形式返回结果。
HtmlHelper类在System.Web.Mvc.Html命令之中,主要由多个静态类组成,它们分别是FormExtensions类、InputExtensions类、LinkExtensions类、SelectExtensions类、TextExtensions类、ValidationExtensions类和RenderPartialExtensions类等。
为了方便开发者使用HtmlHelper类,在视图的WebViewPage类中设置了一个HtmlHelper类型的属性Html,代码如下:
using System;
namespace System.Web.Mvc
{
public abstract class WebViewPage<TModel>: WebViewPage
{
protected WebViewPage();
public AjaxHelper<TModel>Ajax { get; set; }
public HtmlHelper<TModel>Html { get; set; }
public TModel Model { get; }
public ViewDataDictionary<TModel>ViewData { get; set; }
public override void InitHelpers();
protected override void SetViewData(ViewDataDictionary viewData);
}
}
表2-19中列举了HtmlHelper类提供的常用HTML帮助器,使用时,通常调用其静态的方法。
表2-19 HTML帮助器的常用方法

以下为部分HTML帮助器的使用示例。
1.Action
调用指定子操作方法并以HTML字符串形式返回结果,有6个重载方法:
(1)Action(thisHtmlHelperhtmlHelper, stringactionName);
(2)Action(thisHtmlHelperhtmlHelper, stringactionName, objectrouteValues);
(3)Action(this HtmlHelperhtmlHelper, stringactionName, RouteValueDictionary routeValues);
(4)Action(thisHtmlHelperhtmlHelper, stringactionName, stringcontrollerName);
(5)Action(this HtmlHelperhtmlHelper, stringactionName, stringcontrollerName, objectrouteValues);
(6)Action(this HtmlHelperhtmlHelper, stringactionName, stringcontrollerName, RouteValueDictionaryrouteValues);
第4个重载方法的使用示例如下:
<! --开始输出用户信息--> @Html.Action("UserInfo", "User") <! --结束输出用户信息-->
在上述代码中,调用了User控制器中的UserInfo动作,输出的HTML语句是(注意注释部分的内容变化):
<! --开始输出用户信息--> <div> <dl class="dl-horizontal"> <dt>Name</dt> <dd>小明</dd> <dt>Gender</dt> <dd>M</dd> <dt>Birthday</dt> <dd>1981/1/1 0:00:00</dd> </dl> </div> <! --结束输出用户信息-->
2.ActionLink
用于在HTML文档中呈现<a>链接元素,包括10个重载方法。
(1)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName);
(2)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, objectrouteValues);
(3)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, RouteValueDictionaryrouteValues);
(4)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, stringcontrollerName);
(5)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, objectrouteValues, objecthtmlAttributes);
(6)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, RouteValueDictionaryrouteValues, IDictionary<string, object>htmlAttributes);
(7)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, stringcontrollerName, objectrouteValues, objecthtmlAttributes);
(8)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, stringcontrollerName, RouteValueDictionaryrouteValues, IDictionary<string, object>htmlAttributes);
(9)ActionLink(thisHtmlHelperhtmlHelper, stringlinkText, stringactionName, string controllerName, string protocol, string hostName, string fragment, object routeValues, objecthtmlAttributes);
(10)ActionLink(this HtmlHelperhtmlHelper, stringlinkText, stringactionName, stringcontrollerName, stringprotocol, stringhostName, stringfragment, RouteValueDictionary routeValues, IDictionary<string, object>htmlAttributes)。
第4个重载方法的使用示例如下:
@Html.ActionLink("用户管理", "Index", "User")
在上述代码中,调用了User控制器中的Index动作,输出的HTML语句是:
<a href="/User/Index">用户管理</a>
3.TextBox和TextBoxFor
用于在视图中呈现文本框元素<inputtype="text"/>,其中TextBoxFor是针对强类型视图的模型呈现方式。
TextBox方法提供了7个重载方法。
(1)TextBox(thisHtmlHelperhtmlHelper, stringname);
(2)TextBox(thisHtmlHelperhtmlHelper, stringname, objectvalue);
(3)TextBox(thisHtmlHelperhtmlHelper, stringname, objectvalue, IDictionary<string, object>htmlAttributes);
(4)TextBox(this HtmlHelper htmlHelper, string name, objectvalue, object htmlAttributes);
(5)TextBox(this HtmlHelper htmlHelper, string name, objectvalue, string format);
(6)TextBox(this HtmlHelper htmlHelper, string name, objectvalue, string format, IDictionary<string, object>htmlAttributes);
(7)TextBox(this HtmlHelper htmlHelper, string name, objectvalue, string format, objecthtmlAttributes);
TextBoxFor方法提供了6个重载方法。
(1)TextBoxFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression);
(2)TextBoxFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IDictionary<string, object>htmlAttributes);
(3)TextBoxFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, objecthtmlAttributes);
(4)TextBoxFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, stringformat);
(5)TextBoxFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, stringformat, IDictionary<string, object>htmlAttributes);
(6)TextBoxFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty> > expression, string format, object htmlAttributes)。
第2个重载方法的使用示例如下:
@Html.TextBox("Name", "小明")
在上述代码中,生成文本框的名称为Name,默认值为“小明”,输出的HTML语句是:
<input id="Name" name="Name" type="text" value="小明" />
第8个重载方法的使用示例如下:
@Html.TextBoxFor(model =>model.Name)
在上述代码中使用了Lamda,表示与模型中Name属性绑定,输出的HTML与TextBox方法生成的相同。
4.TextArea和TextAreaFor
用于在视图中呈现多行文本框元素<textarea>,其中TextAreaFor是针对于强类型视图的模型呈现方式。
TextArea方法提供了8个重载方法。
(1)TextArea(thisHtmlHelperhtmlHelper, stringname);
(2)TextArea(this HtmlHelperhtmlHelper, stringname, IDictionary<string,object>htmlAttributes);
(3)TextArea(thisHtmlHelperhtmlHelper, stringname, objecthtmlAttributes);
(4)TextArea(thisHtmlHelperhtmlHelper, stringname, stringvalue);
(5)TextArea(thisHtmlHelperhtmlHelper, stringname, stringvalue, IDictionary<string, object>htmlAttributes);
(6)TextArea(this HtmlHelperhtmlHelper, string name, string value, object htmlAttributes);
(7)TextArea(thisHtmlHelperhtmlHelper, stringname, stringvalue, introws, intcolumns, IDictionary<string, object>htmlAttributes);
(8)TextArea(thisHtmlHelperhtmlHelper, stringname, stringvalue, introws, intcolumns, objecthtmlAttributes)。
TextAreaFor方法提供了5个重载方法。
(1)TextAreaFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression);
(2)TextAreaFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IDictionary<string, object>htmlAttributes);
(3)TextAreaFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, objecthtmlAttributes);
(4)TextAreaFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>> expression, introws, intcolumns, IDictionary<string, object>htmlAttributes);
(5)TextAreaFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, introws, intcolumns, object htmlAttributes)。
第4个重载方法的使用示例如下:
@Html.TextArea("Name", "小明")
在上述代码中,生成多行文本框的名称为Name,默认值为“小明”,输出的HTML语句是:
<textarea cols="20" id="Name" name="Name" rows="2">小明</textarea>
第2个重载方法的使用示例如下:
@Html. TextAreaFor(model =>model.Name)
在上述代码中使用了Lamda,表示与模型中Name属性绑定,输出的HTML与TextArea方法生成的相同。
5.Password和PasswordFor
用于呈现输入密码的文本框<inputtype="password"/>,其中PasswordFor是针对于强类型视图的模型呈现方式。
Password方法提供了3个重载方法。
(1)Password(thisHtmlHelperhtmlHelper, stringname, objectvalue);
(2)Password(thisHtmlHelperhtmlHelper, stringname, objectvalue, IDictionary<string, object>htmlAttributes);
(3)Password(this HtmlHelperhtmlHelper, string name, objectvalue, object htmlAttributes);
PasswordFor方法提供了3个重载方法。
(1)PasswordFor< TModel, TProperty>(this HtmlHelper< TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression);
(2)PasswordFor< TModel, TProperty>(this HtmlHelper< TModel>htmlHelper, Expression<Func<TModel, TProperty>> expression, IDictionary<string, object>htmlAttributes);
(3)PasswordFor< TModel, TProperty>(this HtmlHelper< TModel>htmlHelper, Expression< Func< TModel, TProperty> > expression, object htmlAttributes)。
第1个重载方法的使用示例如下:
@Html.Password("Pwd", "123456")
在上述代码中生成了用于输入密码的文本框,名称为Pwd,默认值为“123456”,输出的HTML语句是:
<input id="Pwd" name="Pwd" type="password" value="123456" />
第2个重载方法的使用示例如下:
@Html.PasswordFor(model =>model.Pwd)
在上述代码中使用了Lamda,表示与模型中的Pwd属性绑定,输出的HTML语句是:
<input id="Pwd" name="Pwd" type="password" />
6.RadioButton和RadioButtonFor
它们用于在视图中呈现单选按钮元素<inputtype="radio"/>,其中RadioButtonFor是针对强类型视图的模型呈现方式。
RadioButton方法提供了6个重载方法。
(1)RadioButton(thisHtmlHelperhtmlHelper, stringname, objectvalue);
(2)RadioButton(this HtmlHelperhtmlHelper, stringname, objectvalue, bool isChecked);
(3)RadioButton(this HtmlHelper htmlHelper, string name, object value, IDictionary<string, object>htmlAttributes);
(4)RadioButton(this HtmlHelperhtmlHelper, stringname, objectvalue, object htmlAttributes);
(5)RadioButton(this HtmlHelperhtmlHelper, stringname, objectvalue, bool isChecked, IDictionary<string, object>htmlAttributes);
(6)RadioButton(this HtmlHelperhtmlHelper, stringname, objectvalue, bool isChecked, objecthtmlAttributes);
RadioButtonFor方法提供了3个重载方法。
(1)RadioButtonFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, objectvalue);
(2)RadioButtonFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, objectvalue, IDictionary<string, object>htmlAttributes);
(3)RadioButtonFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, objectvalue, objecthtmlAttributes);
第1个重载方法的使用示例如下:
@Html.RadioButton("Gender", "M")男 @Html.RadioButton("Gender", "F")女
在上述代码中生成了一个选择性别的单选按钮组,名称为Gender,输出的HTML语句是:
<input id="Gender" name="Gender" type="radio" value="M" />男 <input id="Gender" name="Gender" type="radio" value="F" />女
第7个重载方法的使用示例如下:
@Html.RadioButtonFor(model =>model.Gender, "M")男 @Html.RadioButtonFor(model =>model.Gender, "F")女
在上述代码中使用了Lamda,表示与模型中的Gender属性绑定,输出的HTML与RadioButton方法生成的相同。
7.CheckBox和CheckBoxFor
用于向视图中呈现复选按钮元素<inputtype="checkbox"/>,其中CheckBoxFor是针对强类型视图的模型呈现方式。
CheckBox方法提供了6个重载方法。
(1)CheckBox(thisHtmlHelperhtmlHelper, stringname);
(2)CheckBox(thisHtmlHelperhtmlHelper, stringname, boolisChecked);
(3)CheckBox(this HtmlHelperhtmlHelper, stringname, IDictionary<string, object>htmlAttributes);
(4)CheckBox(thisHtmlHelperhtmlHelper, stringname, objecthtmlAttributes);
(5)CheckBox(this HtmlHelper htmlHelper, string name, boolisChecked,IDictionary<string, object>htmlAttributes);
(6)CheckBox(thisHtmlHelperhtmlHelper, stringname, boolisChecked, object htmlAttributes);
CheckBoxFor方法提供了3个重载方法。
(1)CheckBoxFor<TModel>(this HtmlHelper<TModel> htmlHelper, Expression<Func<TModel, bool>>expression);
(2)CheckBoxFor<TModel>(this HtmlHelper<TModel> htmlHelper, Expression<Func<TModel, bool>> expression, IDictionary<string, object>htmlAttributes);
(3)CheckBoxFor<TModel>(this HtmlHelper<TModel> htmlHelper, Expression<Func<TModel, bool>>expression, objecthtmlAttributes);
第6个重载方法的使用示例如下:
@Html.CheckBox("IsSpecialty", true, new { @value ="1" }) Andorid开发 @Html.CheckBox("IsSpecialty", false, new { @value ="2" }) IOS开发 @Html.CheckBox("IsSpecialty", false, new { @value ="3" }) .NET开发 @Html.CheckBox("IsSpecialty", false, new { @value ="4" }) Java开发
在上述代码中生成了一组选择特长的复选按钮组,名称为IsSpecialty,使用htmlAttributes参数为每个多选按钮设置了value值。
<input id="Gender" name="Gender" type="radio" value="M" />男 <input id="Gender" name="Gender" type="radio" value="F" />女
对于第7个重载方法的使用示例如下:
<input id="IsSpecialty" checked="checked" name="IsSpecialty" type= "checkbox" value="1" /><input name="IsSpecialty" type="hidden" value= "false" />Andorid开发 <input id="IsSpecialty" name="IsSpecialty" type="checkbox" value="2" /> <input name="IsSpecialty" type="hidden" value="false" />IOS开发 <input id="IsSpecialty" name="IsSpecialty" type="checkbox" value="3" /> <input name="IsSpecialty" type="hidden" value="false" />.NET开发 <input id="IsSpecialty" name="IsSpecialty" type="checkbox" value="4" /> <input name="IsSpecialty" type="hidden" value="false" />Java开发
在上述代码中使用了Lamda,表示与模型中IsSpecialty属性绑定。需要强调是,使用CheckBoxFor方法绑定的属性的数据类型必须是布尔类型,输出的HTML与CheckBox方法生成的相同。
8.DropDownList和DropDownListFor
用于在视图中呈现下拉列表框元素<select/>,其中DropDownListFor是针对于强类型视图的模型呈现方式。
DropDownList方法提供了8个重载方法。
(1)DropDownList(thisHtmlHelperhtmlHelper, stringname);
(2)DropDownList(this HtmlHelper htmlHelper, string name, IEnumerable<SelectListItem>selectList);
(3)DropDownList(thisHtmlHelperhtmlHelper, stringname, stringoptionLabel);
(4)DropDownList(this HtmlHelper htmlHelper, string name, IEnumerable<SelectListItem>selectList, IDictionary<string, object>htmlAttributes);
(5)DropDownList(this HtmlHelper htmlHelper, string name, IEnumerable<SelectListItem>selectList, objecthtmlAttributes);
(6)DropDownList(this HtmlHelper htmlHelper, string name, IEnumerable<SelectListItem>selectList, stringoptionLabel);
(7)DropDownList(this HtmlHelper htmlHelper, string name, IEnumerable<SelectListItem> selectList, string optionLabel, IDictionary<string, object>htmlAttributes);
(8)DropDownList(this HtmlHelper htmlHelper, string name, IEnumerable<SelectListItem>selectList, stringoptionLabel, objecthtmlAttributes)。
DropDownListFor方法提供了6个重载。
(1)DropDownListFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IEnumerable<SelectListItem>selectList);
(2)DropDownListFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IEnumerable<SelectListItem>selectList, IDictionary<string, object>htmlAttributes);
(3)DropDownListFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IEnumerable<SelectListItem>selectList, objecthtmlAttributes);
(4)DropDownListFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IEnumerable<SelectListItem>selectList, stringoptionLabel);
(5)DropDownListFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IEnumerable<SelectListItem> selectList, string optionLabel, IDictionary<string, object>htmlAttributes);
(6)DropDownListFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression, IEnumerable<SelectListItem>selectList, stringoptionLabel, objecthtmlAttributes)。
第2个重载方法的使用示例如下:
@{ var genderList =new List<SelectListItem>() { new SelectListItem(){ Text="男", Value="M", Selected=true}, new SelectListItem(){ Text="女", Value="F"} }; } @Html.DropDownList("Gender", genderList)
在上述代码中生成了一个下框列表框元素,名称为Gemder,选项内容为IEnumerable<SelectListItem>类型集合。
第9个重载方法使用了Lamda,表示与模型中的Gender属性绑定,代码如下:
@Html.DropDownListFor(model =>model.Gender, genderList)
以上方法生成的HTML内容为:
<select id="Gender" name="Gender"> <option selected="selected" value="M">男</option> <option value="F">女</option> </select>
9.Display和DisplayFor
返回由字符串表达式表示的对象中的每个属性所对应的HTML标记值。
Display方法提供了6个重载方法。
(1)Display(thisHtmlHelperhtml, stringexpression);
(2)Display(thisHtmlHelperhtml, stringexpression, objectadditionalViewData);
(3)Display(thisHtmlHelperhtml, stringexpression, stringtemplateName);
(4)Display(this HtmlHelper html, string expression, string templateName, objectadditionalViewData);
(5)Display(thisHtmlHelperhtml, stringexpression, stringtemplateName, string htmlFieldName);
(6)Display(thisHtmlHelperhtml, stringexpression, stringtemplateName, string htmlFieldName, objectadditionalViewData)。
DisplayFor方法提供了6个重载。
(1)DisplayFor< TModel, TValue>(this HtmlHelper< TModel> html, Expression<Func<TModel, TValue>>expression);
(2)DisplayFor< TModel, TValue>(this HtmlHelper< TModel> html, Expression<Func<TModel, TValue>>expression, objectadditionalViewData);
(3)DisplayFor< TModel, TValue>(this HtmlHelper< TModel> html, Expression<Func<TModel, TValue>>expression, stringtemplateName);
(4)DisplayFor< TModel, TValue>(this HtmlHelper< TModel> html, Expression<Func<TModel, TValue>>expression, stringtemplateName, object additionalViewData);
(5)DisplayFor< TModel, TValue>(this HtmlHelper< TModel> html, Expression<Func<TModel, TValue>>expression, stringtemplateName, string htmlFieldName);
(6)DisplayFor< TModel, TValue>(this HtmlHelper< TModel> html, Expression<Func<TModel, TValue>>expression, stringtemplateName, string htmlFieldName, objectadditionalViewData)。
第1个重载方法的使用示例如下:
姓名:@Html.Display("Name")
在上述代码中,用于显示模型中Name属性对应的HTML值。
第7个重载方法使用了Lamda,表示与模型中的Name属性绑定,显示Name属性对应的值,代码如下。
姓名:@Html. DisplayFor(model =>model.Name)
以上方法生成的HTML内容如下。
姓名:小明
10.BeginForm和BeginRouteForm
用于在视图中呈现表单元素<form/>,使用了3种类型的扩展方法BeginForm、BeginRouteForm及EndForm来完成相关操作。
BeginForm实现表单定义的开始部分,提供了13个重载方法。
(1)BeginForm();
(2)BeginForm(ObjectrouteValues);
(3)BeginForm(RouteValueDictionaryrouteValues);
(4)BeginForm(stringactionName, stringcontrollerName);
(5)BeginForm(stringactionName, stringcontrollerName, objectrouteValues);
(6)BeginForm(stringactionName, stringcontrollerName, RouteValueDictionary routeValues);
(7)BeginForm(stringactionName, stringcontrollerName, FormMethodmethod);
(8)BeginForm(string actionName, string controllerName, objectrouteValues,FormMethodmethod);
(9)BeginForm(stringactionName, stringcontrollerName, RouteValueDictionary routeVaues, FormMethodmethod);
(10)BeginForm(stringactionName, stringcontrollerName, FormMethod method, objecthtmlAttributes);
(11)BeginForm(stringactionName, stringcontrollerName, FormMethod method, IDictionary<string, object>htmlAttributes);
(12)BeginForm(stringactionName, stringcontrollerName, objectrouteValues, FormMethodmethod, objecthtmlAttributes);
(13)BeginForm(stringactionName, stringcontrollerName, RouteValueDictionary routeValues, FormMethodmethod, IDictionary<string, object>htmlAttributes)。
第2个重载方法的使用示例如下:
@Html.BeginForm(new{action="Index", controller="User", id=1});
在上述代码中,设置了路由值的一个实例化对象,输出的HTML语句是:
<form action="User/Index/1" method="post"/>
BeginRouteForm以路由的方法设置action的值,以此实现表单定义的开始部分。提供了12个重载方法:
(1)BeginRouteForm(thisHtmlHelperhtmlHelper, objectrouteValues);
(2)BeginRouteForm(thisHtmlHelperhtmlHelper, RouteValueDictionaryrouteValues);
(3)BeginRouteForm(thisHtmlHelperhtmlHelper, stringrouteName);
(4)BeginRouteForm(this HtmlHelperhtmlHelper, stringrouteName, FormMethod method);
(5)BeginRouteForm(this HtmlHelper htmlHelper, string routeName, object routeValues);
(6)BeginRouteForm(this HtmlHelper htmlHelper, string routeName, RouteValueDictionaryrouteValues);
(7)BeginRouteForm(this HtmlHelperhtmlHelper, stringrouteName, FormMethod method, IDictionary<string, object>htmlAttributes);
(8)BeginRouteForm(this HtmlHelperhtmlHelper, stringrouteName, FormMethod method, objecthtmlAttributes);
(9)BeginRouteForm(this HtmlHelper htmlHelper, string routeName, object routeValues, FormMethodmethod);
(10)BeginRouteForm(this HtmlHelper htmlHelper, string routeName,RouteValueDictionaryrouteValues, FormMethodmethod);
(11)BeginRouteForm(this HtmlHelper htmlHelper, string routeName, object routeValues, FormMethodmethod, objecthtmlAttributes);
(12)BeginRouteForm(this HtmlHelper htmlHelper, string routeName, RouteValueDictionaryrouteValues, FormMethod method, IDictionary<string, object>htmlAttributes)。
第1个重载方法使用示例如下:
@Html.BeginRouteForm(new { controller ="User", action ="Index" })
以上代码通过实例化路由对象的方式指定了controller和action参数,输出的代码如下:
<form action="User/Index" method="post"/>
EndForm作为表单结束部分的标记,代码如下:
@Html.EndForm();
输出的HTML代码为:
</form>
还可以使用using()方法实现结束标记的自动关闭。代码如下:
@using (Html.BeginRouteForm(new { controller ="User", action ="Index" })) { //HTML内容 }
11.Partial和RenderPartial
以HTML的形式呈现指定的分部视图。
Partial方法提供了4个重载方法。
(1)Partial(thisHtmlHelperhtmlHelper, stringpartialViewName);
(2)Partial(thisHtmlHelperhtmlHelper, stringpartialViewName, objectmodel);
(3)Partial(thisHtmlHelperhtmlHelper, stringpartialViewName, ViewDataDictionary viewData);
(4)Partial(this HtmlHelperhtmlHelper, stringpartialViewName, objectmodel, ViewDataDictionaryviewData)。
RenderPartial方法提供了4个重载方法。
(1)RenderPartial(thisHtmlHelperhtmlHelper, stringpartialViewName);
(2)RenderPartial(this HtmlHelperhtmlHelper, stringpartialViewName, object model);
(3)RenderPartial(this HtmlHelper htmlHelper, string partialViewName, ViewDataDictionaryviewData);
(4)RenderPartial(this HtmlHelperhtmlHelper, stringpartialViewName, object model, ViewDataDictionaryviewData)。
第1个重载方法的使用示例如下:
@Html.Partial("_Top")
第5个重载方法的使用示例如下:
@{ Html.RenderPartial("_Top"); }
以上代码均调用名称为“_Top.cshtml”的视图。两个方法的区别在于:Partial方法的返回值为MvcHtmlString,可直接使用@Html.Partial("_Top")方法调用分部视图,而RenderPartial方法的返回值为void,所以需要使用Razor语法的多行语句执行方式调用分部视图。
_Top.cshtml的代码为:
<div>这是页头,当前时间为:@DateTime.Now</div>
调用分部视图后生成的代码为:
<div>这是页头,当前时间为:2016/4/17 22:18:05</div>
12.ValidationMessageFor
用于为由指定表达式表示的每个数据字段的验证错误消息返回对应的HTML标记。该方法提供了4个重载方法。
(1)ValidationMessageFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression<Func<TModel, TProperty>>expression);
(2)ValidationMessageFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression< Func< TModel, TProperty> > expression, string validationMessage);
(3)ValidationMessageFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression< Func< TModel, TProperty> > expression, string validationMessage, IDictionary<string, object>htmlAttributes);
(4)ValidationMessageFor<TModel, TProperty>(this HtmlHelper<TModel>htmlHelper, Expression< Func< TModel, TProperty> > expression, string validationMessage, objecthtmlAttributes)。
第1个重载方法的使用示例如下:
@Html.EditorFor(model =>model.Birthday) @Html.ValidationMessageFor(model =>model.Birthday)
以上代码中的ValidationMessageFor方法用于验证模型中的属性(Birthday),在接受时验证是否符合语义验证的要求,如果不符合,则输出对应的错误信息。输出的HTML代码如下:
<input class="input-validation-error text-box single-line"data-val="true" data-val-date="字段 出生日期 必须是日期。" data-val-regex="日期格式错误" data- val-regex-pattern="^((? :19|20)\d\d)-(0[1-9]|1[012])-(0[1-9]|[12][0-9]|3 [01])$" data-val-required="出生日期 字段是必需的。" id="Birthday" name=" Birthday" type="datetime" value="2033" /> <span class="field-validation-error" data-valmsg-for="Birthday" data- valmsg-replace="true">值“2033”对于 出生日期 无效。</span>
其他更多的HTML帮助器的方法请参考MSDN官网地址:https://msdn.microsoft.com/zh-cn/library/system.web.mvc.html(v=vs.108).aspx。
2.10.2 URL帮助器
URL帮助器使用UrlHelper类提供的方法生成URL,常用的方法如表2-20所示。
表2-20 UrlHelper类的常用方法

以下为部分URL帮助器的使用示例。
1.Action
该方法用于使用指定的操作名称生成操作方法的完全限定URL,有多个重载方法。
(1)stringAction()
(2)stringAction(stringactionName);
(3)stringAction(stringactionName, objectrouteValues);
(4)stringAction(stringactionName, RouteValueDictionaryrouteValues);
(5)stringAction(stringactionName, stringcontrollerName);
(6)stringAction(stringactionName, stringcontrollerName, objectrouteValues);
(7)string Action(string actionName, string controllerName, RouteValueDictionary routeValues);
(8)stringAction(stringactionName, stringcontrollerName, objectrouteValues, stringprotocol);
(9)string Action(string actionName, string controllerName, RouteValueDictionary routeValues, stringprotocol);
(10)string Action(stringactionName, stringcontrollerName, RouteValueDictionary routeValues, stringprotocol, stringhostName)。
第3个重载方法的使用示例如下:
<a href="@Url.Action("Index", new { name="xiaoming" })">查询</a>
生成的HTML代码为:
<a href="/User/Index? name=xiaoming">查询</a>
2.Content
该方法用于将虚拟(相对)路径转换为应用程序的绝对路径,方法如下:
string Content(string contentPath);
调用示例如下:
<a href="@Url.Content("~/User/Index")">查询</a>
生成的HTML代码为:
<a href="/User/Index">查询</a>
3.HttpRouteUrl
该方法用于返回一个包含内容URL的字符串,有2个重载方法:
(1)stringHttpRouteUrl(stringrouteName, objectrouteValues);
(2)stringHttpRouteUrl(stringrouteName, RouteValueDictionaryrouteValues)。
第1个重载方法的使用示例如下:
< a href="@Url.HttpRouteUrl("Default", new {controller="User", action=" Index", id=2})">查询</a>
以上方法指定了路由名称为Default,并指定了路由参数controller="User", action="Index", id=2,生成的HTML代码为:
<a href="/User/Index/2? httproute=True">查询</a>
4.Encode
该方法用于将URL字符串中的特殊字符编码为字符实体等效项,通常在URL中有中文字符时使用。方法为:
string Encode(string url);
示例代码如下:
<a href="@Url.Encode("/User/Index? name=小明")">查询</a>
以上代码会对URL中的字符重新编码,生成的HTML代码为:
<a href="% 2fUser% 2fIndex% 3fname% 3d% e5% b0% 8f% e6% 98% 8e">查询</a>