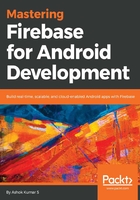
上QQ阅读APP看书,第一时间看更新
Structuring the data with objects
Create a model class with constructors and declare a string to fetch the database reference for a unique key to add the list of objects. The model class is as follows:
public class Users {
private String Name;
private String Email;
private String Phone;
public Users() {
}
public String getName() {
return Name;
}
public void setName(String name) {
Name = name;
}
public String getEmail() {
return Email;
}
public void setEmail(String email) {
Email = email;
}
public String getPhone() {
return Phone;
}
public void setPhone(String phone) {
Phone = phone;
}
public Users(String name, String email, String phone) {
Name = name;
Email = email;
Phone = phone;
}
}
Now in the activity class using the DatabaseReference class we can set the object value to Firebase, as follows:
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private FirebaseDatabase mDatabase;
private DatabaseReference mDbRef;
private String userId;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Write a message to the database
mDatabase = FirebaseDatabase.getInstance();
mDbRef = mDatabase.getReference("Donor/Name");
//Setting firebase unique key for Hashmap list
String userId = mDbRef.push().getKey();
// creating user object
Users user = new Users("Hillary", "hillary@xyz.com", "90097863873", "Tokyo");
mDbRef.child(userId).setValue(user);
}
}
The preceding code will add the object into Firebase as follows:
