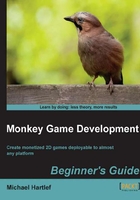
Time for action — acting on the different game modes
The last thing to add is the need to act to the game modes. We have three modes:
- 0=Start of game
- 1=Gameplay
- 2=GameOver
We want to direct and inform the player about what mode the game is in and how they can start it. Then if the victory conditions are achieved, we want to give a visual response.
- The first mode is bound to a new method that we will create now. Create a new method called
StartGame
.Method StartGame:Int()
- We want to print a message only once; that is why we need the field
modeMessage
. If it is0
, then we can print this message. If we don't use such an approach, the message will be printed every time we call this method.If modeMEssage = 0 Then
- Now set
messageMode
to1
and print a message:modeMessage = 1 Print ("Press P to start the game") Endif
- In this method, we will also check if the
P
key was hit and setmessageMode
andgameMode
accordingly.If KeyHit(KEY_P) Then modeMessage = 0 gameMode = 1 'mode = Game playing Endif
- Close off the method now.
Return True End
The method for
gameMode = 1
exists already; it's theUpdateGame
method we have created and modified before.For
gameMode = 2
, we will need another method that informs the player about the end of the game and who the winner is. - Create a new method called
GameOver
.Method GameOver_Int()
- Again, we will print some messages now, and we want to do this only once. Remember this method will be called at each frame as long we are in this game mode. So we check against
modeMessage
=0
now.If modeMessage = 0 Then
- Now, set
modeMessage
to1
and print an info message that the game has ended.modeMessage = 1 Print ("G A M E O V E R")
- Depending on who has more points, we will inform the player who won the game. For this, we will check if
ePoints
is equal to or greater than10
.If ePoint >= 10 Then Print ("Don't cry, the computer won! Next time try harder.") Else Print ("Congratulations, you won! It must be your lucky day.") Endif
- We want to inform the player now about what they can do next and close off this
IF
check.Print ("Press P to restart the game") Endif
- As the player has the ability to restart the game, we will check now whether the
P
key was hit. Then, we will set thegameMode
variable accordingly and also some position variables.If KeyHit(KEY_P) Then ePoints = 0 pPoints = 0 Print (ePoints + ":" + pPoints) pY = 240.0 'player paddle Y pos bX = 320.0 'ball Y pos bY = 240.0 'ball Y pos bdX = 3.5 'ball X speed bdY = 1.5 'ball Y speed eY[0] = 240.0 'enemy paddle 1 Y pos eY[1] = 240.0 'enemy paddle 2 Y pos modeMessage = 0 gameMode = 1 Endif
- Close off this method now.
Return True End
- Not much is left to building our game. We have built the last two methods for the missing game modes and now call them when we need to. For this, we need to replace the
OnUpdate
method.Method OnUpdate:Int()
- To check which mode the game is in, we will use a
Select
statement this time and call the different methods according to the value of the fieldgameMode
.Select gameMode Case 0 StartGame() Case 1 UpdateGame() Case 2 GameOver() End
- Close off our new
OnUpdate
method, now.Return True End
- Save the game one last time and test it. For sure, the enemy paddles are not very competitive, but it will be a good practice for you to enhance this. If you want to compare your code to a final version, load up
Pongo_final.Monkey
.
What just happened?
Yeah, you can be proud of yourself. You have coded your first little game. It has everything a good game needs—good data structure, separate render and update methods, even collision detection, and a very simple computer AI.
Have a go hero — enhancing the computer AI
It will be good practice for you if you try to make the enemy paddles react more intelligently. Try to do this, perhaps as follows:
- Maybe they should react on the Y speed of the ball somehow
- Make the start of the ball more random
- Give it a try and don't be afraid to change this. Just remember... save your code!