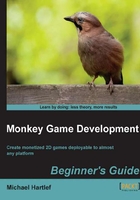
Time for action — building the basic file structure of the game
Let's begin with the basic game structure:
- Open an empty script and save it under the file name
RocketCommander.monkey
, in a folder of your choice. - The first line in this new script will tell Monkey to compile your script in
STRICT
mode.Strict
- Now, add some comments about the script.
#rem Script: RocketCommander.monkey Description: Sample script from chapter #3 of the book "Monkey Game Development Beginners guide" by PacktPub Author: Michael Hartlef #end
- This time we won't import mojo, but another file, in which we'll import all the classes and other files. This file will be created soon, under the name
gameClasses.monkey
.Import gameClasses
- And finally in this file, we need the
main
function. Without it, Monkey will complain when you try to build it.Function Main:Int()
- Inside the
main
function, we will create a new instance of the main class, calledRocketCommander
, and store it inside a global variable calledgame
, which again will be created very soon in another file.game = New RocketCommander
- Now, close the function.
Return True End
- Ok, that is all for this file. Save it.
You might close it for now, but when you want to run the game later, this is the file you want to build from.
As you can see, this is quite an unusual approach, as we have used identifiers and objects which don't exist at the moment. But, you will create them very soon! First, we start with creating the file to import all the classes, functions, and data definitions.
- Create an empty file and save it in the same folder as the first one, with the name
gameClasses.monkey
. - In this empty script, tell Monkey to use the
Strict
mode again (for this file and importing the mojo framework).Strict Import mojo
- In this file, we will also import our other files that include all the classes, functions, and global definitions. Add an
Import
command for the next file we will create.Import mainClass
- Now save the
gameClasses.monkey
file and close it for now.The next file we will create is called
mainClass.monkey.
This will store our main class,RocketCommander
. - Create a new empty script and save it under the name
mainClass.monkey
. - Tell Monkey to activate the
Strict
mode in this file and import the previously created filegameClasses.monkey
.Strict Import gameClasses
- As we have mentioned a variable called
game
before, we will add its definition to this file now. Remember,game
holds the instance of the classRocketCommander
.Global game:RocketCommander
- Now, we create the actual
RocketCommander
class, which of course extends from mojo'sApp
class.Class RocketCommander Extends App
As usual, we will add the
OnCreate, OnUpdate
, andOnRender
methods. - Let's start with the
OnCreate
method. Remember that it will always have to contain aSetUpdateRate
statement.Method OnCreate:Int() SetUpdateRate(60) 'Set update rate to 60 fps Return True End
- Next, we will add the
OnUpdate
andOnRender
methods.Method OnUpdate:Int() Return True End Method OnRender:Int() Return True End
- It is time to close this class.
Return True End
- Save the file and close it for now.
What just happened?
We have created three files for now. The RocketCommander.monkey
file holds the Main
function and is the one that has to be built when you want to compile the game. The gameClasses.monkey
file imports all other files where we actually defined the game. It's also the only file we will import in all other files. And last but not least, we have created our mainClass.monkey
file, which will hold the main class called RocketCommander
.
You could try to build and run the game now. If Monkey does not report any problems, you should see just a white screen in the browser. So let's get closer to something visible.