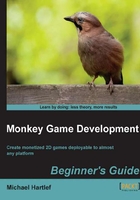
Time for action — implementing the rocket launchers
As you will see yourself, quite a few things look similar to the city
class definition and its wrapper functions. However, the launchers are different objects, and so we need different methods and functions for them. Not every step of code is explained now, as some is the same as for the city
class.
- Create an empty script and save it under the name
launcherClass.monkey
. - At the beginning of the script, tell Monkey to switch into
Strict
mode and import thegameClasses.monkey
file.Strict Import gameClasses
- Create a new list that stores all instances of the
launcher
class.Global launchers := New List<launcher>
Launchers will have to be updated for each frame, so we will have a new wrapper function to call the corresponding method for all existing launchers.
- Create a wrapper function called
UpdateLaunchers
. In it, we will loop through the launcher's list and call theirUpdate
method.Function UpdateLaunchers:Int() 'Loop through the list of launchers For Local launcher := Eachin launchers 'Call the Update method of each launcher launcher.Update() Next Return True End
The same goes here with drawing the launchers to the canvas.
- Add a function called
RenderLaunchers
. In it, we will loop through the launcher's list to call theirRender
method.Function RenderLaunchers:Int() For Local launcher := Eachin launchers launcher.Render() Next Return True End
- At some point, we will want to know how many rockets are left totally in the game. So, create a new function called
GetTotalRocketcount
. In this function, we will add up all the rockets from each launcher found.Function GetTotalRocketcount:Int() Local rc:Int = 0 'Var to store the total amount of rockets 'Loop through the list of launchers For Local launcher := Eachin launchers 'Add the rocket count to the global var rc rc += launcher.cRockets Next Return rc 'Return back the number of rockets found. End
To add rockets to to each launcher, we will build another function that does just that.
- Add a function called
AddLauncherRockets
. As a parameter, it will take the amount, which then will be added to a launcher inside the loop.Function AddLauncherRockets:Int(ramount:Int) For Local launcher := Eachin launchers launcher.cRockets += ramount 'Add rockets to the launcher Next Return True End
Now, we will add a function to actually create a rocket launcher.
- The function you will now add will get the name
CreateLauncher
. As parameters, it will have thex
position of the launcher, the keyboard key that triggers a shot, and the number of rockets it has initially.Function CreateLauncher:Int(xpos:Float, triggerkey:Int, rcount:Int) Local l:launcher = New launcher 'Create a new launcher l.Init(xpos, triggerkey, rcount) 'Initialize it launchers.AddLast(l) 'Add the launcher to the launchers list Return True End
The last wrapper function we will add is to remove all existing launchers.
- Add a function called
RemoveLaunchers
. In it, we will call theClear
method of the launchers list.Function RemoveLaunchers:Int() launchers.Clear() Return True End
Phew! Again, quite a few wrapper functions! But now it is time for the actual
launcher
class. - Create a new class called
launcher
.Class launcher
- It will feature four fields for the position, the rocket count, and a trigger key.
Field x:Float = 0.0 'x pos of a launcher Field y:Float = 0.0 'y pos of a launcher Field cRockets:Int = 0 'Rocket count Field triggerKey:Int = 0 'Trigger key to shoot
To initialize a launcher, the class has an
Init
method. - Add a method called
Init
, parameters for thex
position, the trigger key, and the count of rockets.Method Init:Int(xpos:Float, trigkey:Int, rcount:Int) x = xpos 'Set the x position y = game.cHeight - 40.0 'Calculate the y position cRockets = rcount 'Set the rocket count triggerKey = trigkey 'Set the trigger key Return True End
Updating a launcher means checking if the trigger key was pressed and then launching a rocket at the target.
- To update a launcher, add the
Update
method.Method Update:Int()
- Check if the trigger key was hit and that there are still rockets left.
If KeyHit(triggerKey) And cRockets > 0 Then
- The next function call is one that doesn't exist at the moment.
CreateRocket
will be featured in the next class that we add. Still, add it as a comment.'CreateRocket(MouseX(), MouseY(), Self.x )
- Reduce the rocket count and close off the IF check and the method.
cRockets -= 1 Endif Return True End
Again, as with the
city
class, we have arender
method here too. After all, we need to render the launchers, right? - Add a
Render
method.Method Render:Int()
- First, we set the color to turquoise. Then, we draw a circle for the launcher head and a rectangle for the launcher base.
SetColor(0, 255, 255) DrawCircle(x, y - 15, 5) DrawRect(x-2, y - 15, 4, 15)
- Next, we will draw some markers for the remaining rockets. Start with setting the color to white.
SetColor(255, 255, 255)
- Next, we will calculate the local
xdiff
variable that will be an offset, to draw the rocket markers.Local xdiff:Int = cRockets*3 / 2
- Now, check if some rockets are left.
If cRockets > 0 Then
- For each rocket, we will draw a small line beneath the launcher.
For Local i:Int = 1 To cRockets DrawLine(x + i*3 - xdiff, y+20, x + i*3 - xdiff, y + 30) Next Endif
- Close the method and the class.
Return True End End
We're done! The launcher class is ready to be implemented.
What just happened?
Now we have the device to fire our rockets. Sadly there aren't any rockets to fire, but we will get there. But what have we done? We built a class for a launcher with its own Init, Update
, and Render
methods. Also, we created some wrapper functions that will help us to act on several launchers at once. The class is ready to be implemented; the only thing we have to change later on is a small line in the Update
method, so that it becomes active:
CreateRocket(MouseX(), MouseY(), Self.x )