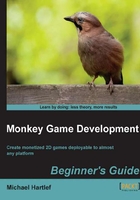
上QQ阅读APP看书,第一时间看更新
Time for action — implementing explosions
To implement explosions follow the ensuing steps:
- Create a new script and save it under the name
explosionClass.monkey
. - Again, switch into
Strict
mode, importgameClasses
, and create a global list calledexplosions
, to store each explosion created in it.Strict Import gameClasses Global explosions := New List<explosion>
Just like with the other objects before, we need some wrapper functions, which we can implement into the
RocketCommander
class. Only the names of the functions are different, so you already now how they work. - Implement functions to create, update, render, and remove explosions.
Function UpdateExplosions:Int() For Local explosion := Eachin explosions explosion.Update() Next Return True End Function RenderExplosions:Int() For Local explosion := Eachin explosions explosion.Render() Next Return True End Function RemoveExplosions:Int() explosions.Clear() Return True End Function CreateExplosion:Int(x:Float, y:Float) Local e:explosion = New explosion e.Init(x, y) explosions.AddLast(e) Return True End
Now, we will add the actual
explosion
class. - Add a new class called
explosion
.Class explosion
- To store the position of an explosion, we need the usual fields.
Field x:Float = 0.0 'x pos of an explosion Field y:Float = 0.0 'y pos of an explosion
- As an explosion is a circle, we need fields to store the radius.
Field cr:Float = 2.0 'current radius Field tr:Float = 40.0 'target radius
- The last field will be a score modifier, which will be used when a bomb collides with the explosion and we get points for it.
Field score:Int = 100 'Points for destroying a bomb
Now, let's add some methods so that the class is functional.
- Add methods to initialize, render, and update an explosion.
Method Init:Int(xp:Float,yp:Float) x = xp y = yp Return True End Method Update:Int() cr += 0.25 'Raise the radius 'Check if target radius is reached If cr > tr Then explosions.Remove(Self) Endif Return True End Method Render:Int() Local cd:Int = Rnd(0,100) SetColor(255-cd, 155+cd, 0) DrawCircle(x, y, cr) Return True End End
What just happened?
We have created a class that deals with explosions. The explanation to implement it was very short as we did similar things with cities, launchers, and rockets, before. There is no need to repeat everything again.